Das sieht auf dem Oszi jetzt mit dem Code:
Code:
void getIR(void)
{
I2CTWI_transmitByte_RepeatedStart(0x55<<1,0x07);
uint8_t irmsb = I2CTWI_readByte(0x55<<1);
//[...] Weitere Bearbeitung der Variablen, keine weiteren Abfragen
}
so aus:
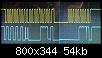
Das sind aber keine zwei Starts, oder?
Grüße
teamohnename
EDIT:
Müsste man also bei task_i2c eine neue else if Abfrage und einen neuen TWI TRANSMISSION STATE hinzufügen, der dann in der neuen Frage abgefragt wird? Also z.B.
Code:
//in RP6I2CMasterTWI.h
#define I2CTWI_REQUEST_BYTES_REP_START 4
//in task_i2c
else if (TWI_operation == I2CTWI_REQUEST_BYTES_REP_START) {
no_rep_start = 1;
I2CTWI_delay();
TWI_msgSize = I2CTWI_request_size + 1;
I2CTWI_request_adr = I2CTWI_request_adr | TWI_READ;
I2CTWI_buf[0] = I2CTWI_request_adr | TWI_READ;
TWI_statusReg.all = 0;
TWCR = (1<<TWEN)|(1<<TWIE)|(1<<TWINT)|(0<<TWEA)|(0<<TWSTA)|(0<<TWSTO);
TWI_operation = I2CTWI_READ_BYTES_FROM_BUFFER;
}
Wo muss dann TWI_operation auf 4 gesetzt werden? In einer Read Funktion?
EDIT2:
Wir kommen gerade überhaupt nicht weiter... Wir glauben auch nicht wirklich, dass das irgendetwas mit einem zweiten Start zu tun haben könnte...
Was muss jetzt genau wo ergänzt werden? Ist wenigstens unser Ansatz richtig?
Sorry für die Nerverei... Aber anders kommen wir nicht weiter, die ganze RP6 Lib ist ohne diese Erklärungen viel zu komplex für uns...
EDIT3:
Unser task_i2c sieht bis jetzt so aus:
Alles Fett gedruckte haben wir geändert.
Code:
void task_I2CTWI(void)
{
if (!I2CTWI_isBusy()) {
if (TWI_statusReg.lastTransOK) {
if(TWI_operation) {
if(TWI_operation == I2CTWI_SEND_REGISTER) {
I2CTWI_delay();
TWI_msgSize = 2;
I2CTWI_buf[0] = I2CTWI_request_adr;
I2CTWI_buf[1] = I2CTWI_request_reg;
TWI_statusReg.all = 0;
TWCR = (1<<TWEN)|(1<<TWIE)|(1<<TWINT)|(0<<TWEA)|(1<<TWSTA)|(0<<TWSTO);
if(no_rep_start == 1)
{
TWI_operation = I2CTWI_REQUEST_BYTES;
}
else
{
TWI_operation = I2CTWI_REQUEST_BYTES_REP_START;
}
}
else if (TWI_operation == I2CTWI_REQUEST_BYTES_REP_START) {
I2CTWI_delay();
TWI_msgSize = I2CTWI_request_size + 1;
I2CTWI_request_adr = I2CTWI_request_adr | TWI_READ;
I2CTWI_buf[0] = I2CTWI_request_adr | TWI_READ;
TWI_statusReg.all = 0;
no_rep_start = 1;
TWCR = (1<<TWEN)|(1<<TWIE)|(1<<TWINT)|(0<<TWEA)|(0<<TWSTA)|(0<<TWSTO);
TWI_operation = I2CTWI_READ_BYTES_FROM_BUFFER;
}
else if (TWI_operation == I2CTWI_REQUEST_BYTES) {
I2CTWI_delay();
TWI_msgSize = I2CTWI_request_size + 1;
I2CTWI_request_adr = I2CTWI_request_adr | TWI_READ;
I2CTWI_buf[0] = I2CTWI_request_adr | TWI_READ;
TWI_statusReg.all = 0;
TWCR = (1<<TWEN)|(1<<TWIE)|(1<<TWINT)|(0<<TWEA)|(1<<TWSTA)|(0<<TWSTO);
TWI_operation = I2CTWI_READ_BYTES_FROM_BUFFER;
}
else if (TWI_operation == I2CTWI_READ_BYTES_FROM_BUFFER) {
TWI_operation = I2CTWI_NO_OPERATION;
if(I2CTWI_requestID!=-1)
I2CTWI_requestedDataReadyHandler(I2CTWI_requestID);
}
}
}
else {
uint8_t errState = I2CTWI_getState();
if(errState != 0) {
TWI_operation = I2CTWI_NO_OPERATION;
TWI_statusReg.lastTransOK = 1;
I2CTWI_request_adr = 0;
I2CTWI_requestID = 0;
I2CTWI_request_size = 0;
I2CTWI_transmissionErrorHandler(errState);
}
}
}
}
Das ist unsere Transmit Funktion:
Code:
void I2CTWI_transmitByte_RepeatedStart(uint8_t targetAdr, uint8_t data)
{
while(I2CTWI_isBusy() || TWI_operation != I2CTWI_NO_OPERATION) task_I2CTWI();
I2CTWI_delay();
TWI_msgSize = 2;
I2CTWI_buf[0] = targetAdr;
I2CTWI_buf[1] = data;
TWI_statusReg.all = 0;
no_rep_start = 0;
TWCR = (1<<TWEN)|(1<<TWIE)|(1<<TWINT)|(0<<TWEA)|(1<<TWSTA)|(0<<TWSTO);
}
Und das unsere Read Funktion:
Code:
uint8_t I2CTWI_readByte(uint8_t targetAdr)
{
while(I2CTWI_isBusy() || TWI_operation != I2CTWI_NO_OPERATION) task_I2CTWI();
I2CTWI_delay();
if(no_rep_start == 1)
{
TWI_operation = I2CTWI_REQUEST_BYTES;
}
else
{
TWI_operation = I2CTWI_REQUEST_BYTES_REP_START;
}
I2CTWI_request_adr = targetAdr;
I2CTWI_requestID = -1;
I2CTWI_request_size = 1;
while(TWI_operation != I2CTWI_NO_OPERATION) task_I2CTWI();
if (TWI_statusReg.lastTransOK)
return I2CTWI_recbuf[1];
else
return 0;
}
Die I2C ISR sieht so aus:
Code:
uint8_t no_rep_start; //Wird ein Repeated start vom Slave benötigt?
ISR (TWI_vect)
{
static uint8_t TWI_bufPos = 0;
switch (TWSR)
{
case TWI_START: // START has been transmitted
case TWI_REP_START: // Repeated START has been transmitted
TWI_bufPos = 0; // Set buffer pointer to the TWI Address location
case TWI_MTX_ADR_ACK: // SLA+W has been transmitted and ACK received
case TWI_MTX_DATA_ACK: // Data byte has been transmitted and ACK received
if (TWI_bufPos < TWI_msgSize) {
TWDR = I2CTWI_buf[TWI_bufPos++];
TWCR = (1<<TWEN)| // TWI Interface enabled
(1<<TWIE)|(1<<TWINT)| // Enable TWI Interupt and clear the flag to send byte
(0<<TWEA)|(0<<TWSTA)|(0<<TWSTO)| //
(0<<TWWC); //
} else { // Send STOP after last byte
TWI_statusReg.lastTransOK = 1; // Set status bits to completed successfully.
if(no_rep_start == 1){
TWCR = (1<<TWEN)| // TWI Interface enabled
(0<<TWIE)|(1<<TWINT)| // Disable TWI Interrupt and clear the flag
(0<<TWEA)|(0<<TWSTA)|(1<<TWSTO)| // Initiate a STOP condition.
(0<<TWWC); //
}
else{
TWCR = (1<<TWEN)| // TWI Interface enabled
(0<<TWIE)|(1<<TWINT)| // Disable TWI Interrupt and clear the flag
(0<<TWEA)|(1<<TWSTA)|(0<<TWSTO)| // Initiate a REPEATED START condition.
(0<<TWWC); //
}
}
break;
case TWI_MRX_DATA_ACK: // Data byte has been received and ACK transmitted
I2CTWI_recbuf[TWI_bufPos++] = TWDR;
case TWI_MRX_ADR_ACK: // SLA+R has been transmitted and ACK received
if (TWI_bufPos < (TWI_msgSize-1) ) { // Detect the last byte to NACK it.
TWCR = (1<<TWEN)| // TWI Interface enabled
(1<<TWIE)|(1<<TWINT)| // Enable TWI Interupt and clear the flag to read next byte
(1<<TWEA)|(0<<TWSTA)|(0<<TWSTO)| // Send ACK after reception
(0<<TWWC); //
} else { // Send NACK after next reception
TWCR = (1<<TWEN)| // TWI Interface enabled
(1<<TWIE)|(1<<TWINT)| // Enable TWI Interupt and clear the flag to read next byte
(0<<TWEA)|(0<<TWSTA)|(0<<TWSTO)| // Send NACK after reception
(0<<TWWC); //
}
break;
case TWI_MRX_DATA_NACK: // Data byte has been received and NACK transmitted
I2CTWI_recbuf[TWI_bufPos] = TWDR;
TWI_statusReg.lastTransOK = 1; // Set status bits to completed successfully.
TWCR = (1<<TWEN)| // TWI Interface enabled
(0<<TWIE)|(1<<TWINT)| // Disable TWI Interrupt and clear the flag
(0<<TWEA)|(0<<TWSTA)|(1<<TWSTO)| // Initiate a STOP condition.
(0<<TWWC); //
break;
case TWI_ARB_LOST: // Arbitration lost
TWI_TWSR_state = TWSR; // Store TWSR
TWCR = (1<<TWEN)| // TWI Interface enabled
(1<<TWIE)|(1<<TWINT)| // Enable TWI Interupt and clear the flag
(0<<TWEA)|(1<<TWSTA)|(0<<TWSTO)| // Initiate a (RE)START condition.
(0<<TWWC); //
break;
default:
TWI_TWSR_state = TWSR; // Store TWSR
TWCR = (1<<TWEN)| // Enable TWI-interface and release TWI pins
(0<<TWIE)|(1<<TWINT)| // Disable Interupt
(0<<TWEA)|(0<<TWSTA)|(1<<TWSTO)| // No Signal requests
(0<<TWWC);
break;
}
}
Und so unsere Funktion zum Abfragen des Sensors:
Code:
void getIR(void)
{
I2CTWI_transmitByte_RepeatedStart(0x55<<1,0x07);
uint8_t irmsb = I2CTWI_readByte(0x55<<1);
}
So sieht das auf dem Oszilloskop aber immer noch aus, wie bei dem Bild in diesem Post oben.
Was muss noch geändert werden?
Lesezeichen